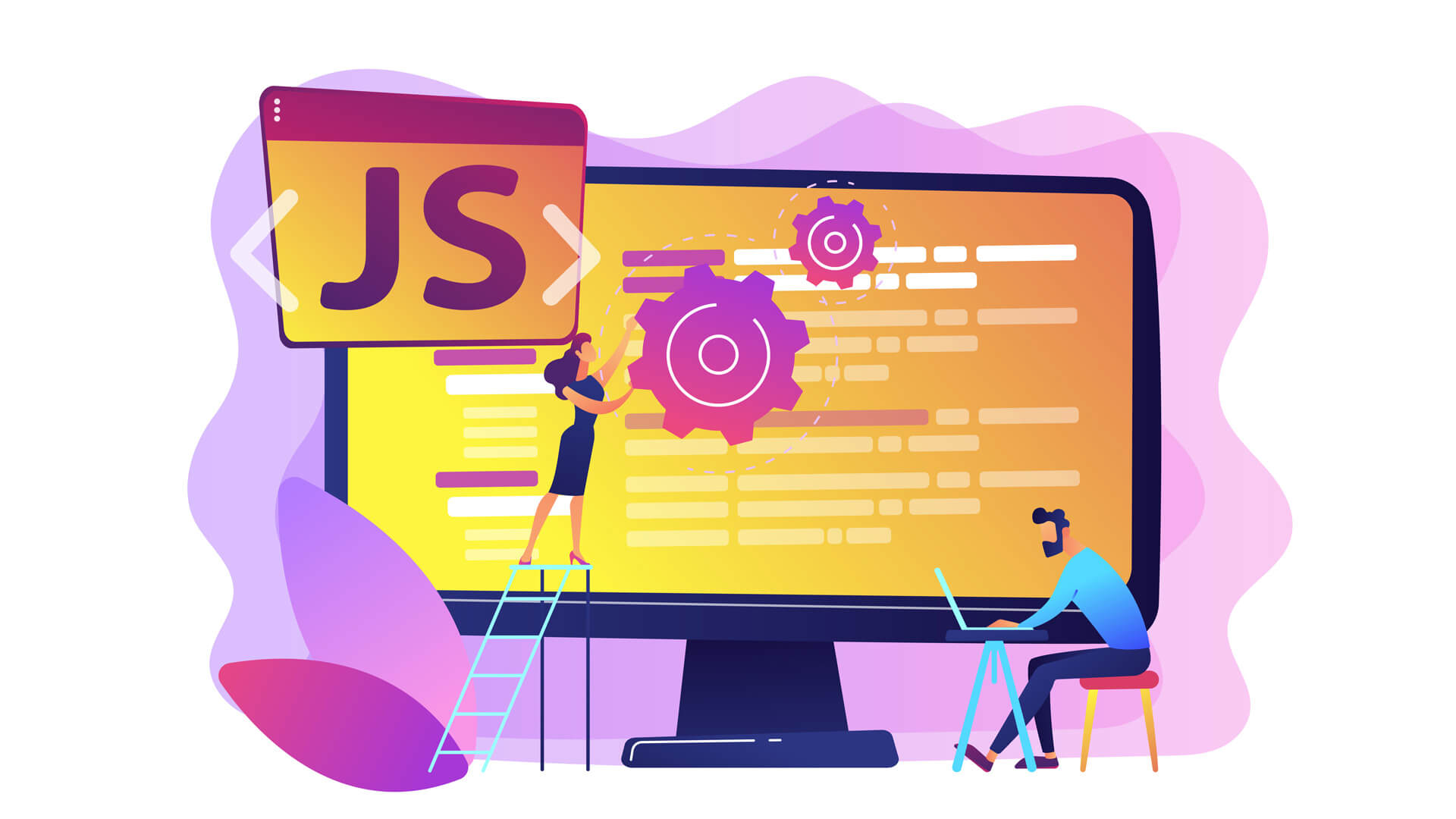
JavaScript is constantly evolving, introducing new features that simplify and speed up frontend development. To stay up-to-date, you need to know and understand these functionalities. In this article, I will introduce you to the 10 most important JavaScript features that every modern frontend developer should master.
Table of Contents
- Arrow Functions – Short and Concise
- Template Literals – More Than Just Strings
- Destructuring Assignment – Elegant Variable Assignment
- Default Parameters – Error-Free Function Handling
- Rest and Spread Operators – Pure Flexibility
- Async/Await – Simplified Asynchronous Programming
- Optional Chaining – Safely Navigating Through Objects
- Nullish Coalescing Operator – Safe Value Assignment
- JavaScript-Modules – Structured and Maintainable Code
- Promise.allSettled – Waiting for All Promises Independently
1. Arrow Functions – Short and Concise
Arrow Functions are one of the most important improvements introduced with ES6 (ECMAScript 2015). They allow for a shorter and more concise syntax for function definitions while also retaining the value of this
from the surrounding context.
// Traditional Function
function add(a, b) {
return a + b;
}
// Arrow Function
const add = (a, b) => a + b;
Why Are Arrow Functions Important? They not only make the code more readable but are also particularly useful when working with callbacks, such as in map
, filter
, or reduce
functions. The shorter syntax allows you to make the code more concise and cleaner in many cases.
2. Template Literals – More Than Just Strings
Template Literals make working with strings much more convenient. Instead of tediously concatenating strings with plus signs, you can directly insert variables and expressions into the string.
const name = "Anna";
const message = `Hello, my name is ${name}.`;
console.log(message); // "Hello, my name is Anna."
With Template Literals, you can also create multiline strings without having to awkwardly concatenate strings. This is especially helpful when creating HTML content or complex strings.
3. Destructuring Assignment – Elegant Variable Assignment
Destructuring allows you to quickly and easily extract values from arrays or objects into individual variables. This saves time and reduces the number of lines in your code.
const person = { name: "John", age: 30 };
const { name, age } = person;
console.log(name); // "John"
console.log(age); // 30
Destructuring not only helps keep your code cleaner but also allows you to handle complex structures more efficiently, which is particularly useful when working with API data.
4. Default Parameters – Error-Free Function Handling
Imagine you have a function that is sometimes called with an argument and sometimes without. Instead of manually checking if a value is present, you can use Default Parameters.
function greet(name = "Guest") {
return `Hello, ${name}!`;
}
console.log(greet()); // "Hello, Guest!"
Default Parameters help you avoid errors and make your code more precise and robust.
5. Rest and Spread Operators – Pure Flexibility
The Spread Operator (...
) and the Rest Operator are versatile tools in JavaScript. They allow you to manipulate arrays and objects in various ways.
const nums = [1, 2, 3];
const moreNums = [...nums, 4, 5];
console.log(moreNums); // [1, 2, 3, 4, 5]
function sum(...numbers) {
return numbers.reduce((a, b) => a + b, 0);
}
console.log(sum(1, 2, 3, 4)); // 10
These operators are extremely useful when working with variable argument lists or when you want to clone or combine data structures.
6. Async/Await – Simplified Asynchronous Programming
Async/Await revolutionizes the way we write asynchronous code. It provides a simpler and more intuitive alternative to Promises, making the code more readable and easier to maintain.
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error retrieving the data:', error);
}
}
By using async
and await
, the code appears synchronous even though it is executed asynchronously. This simplifies error handling and makes the programming logic clearer.
7. Optional Chaining – Safely Navigating Through Objects
With optional chaining (?.
), you can access nested object properties without triggering an error if a property is undefined
or null
.
const user = { profile: { name: "Alice" } };
console.log(user.profile?.name); // "Alice"
console.log(user.address?.street); // undefined
Optional Chaining prevents errors in your code and saves you from many manual checks.
8. Nullish Coalescing Operator – Safe Value Assignment
The Nullish Coalescing Operator (??
) provides a safe way to define default values when the original value is null
or undefined
.
const input = null;
const value = input ?? "Standardvalue";
console.log(value); // "Standardvalue"
This makes your code more robust and ensures that unexpected null
values are handled correctly.
9. JavaScript Modules – Structured and Maintainable Code
JavaScript modules allow you to break your code into smaller, more manageable parts. This not only improves readability but also makes code reuse easier.
// File: math.js
export function add(a, b) {
return a + b;
}
// File: main.js
import { add } from './math.js';
console.log(add(2, 3)); // 5
By using modules, your project remains organized and maintainable, especially in larger applications.
10. Promise.allSettled – Waiting for All Promises Independently
With Promise.allSettled
, you can ensure that you check the status of all promises, regardless of whether they were fulfilled or rejected.
const promises = [Promise.resolve(1), Promise.reject('Error'), Promise.resolve(3)];
Promise.allSettled(promises).then(results => results.forEach(result => console.log(result)));
This is especially useful when working with multiple asynchronous operations and you want to handle the results independently.
Keeping an Eye on the Future of JavaScript Development
These 10 JavaScript features are essential for modern frontend development and help you write more efficient, readable, and robust code. By mastering these features, you can not only accelerate the development process but also create more maintainable applications. The ongoing evolution of JavaScript continuously offers new possibilities, making it important to stay updated and integrate new features into your workflow.
Frequently Asked Questions (FAQ)
Why are Arrow Functions so important?
Arrow Functions provide a compact syntax and inherit the this
value from the surrounding context. This makes the code shorter, more readable, and especially useful when working with callbacks.
How do the Spread and Rest Operators work?
The Spread Operator (...
) allows you to copy or combine arrays and objects, while the Rest Operator can split variables into arrays or objects. Both operators enhance the flexibility and readability of the code.
What advantages does Async/Await offer over Promises?
Async/Await makes asynchronous code simpler to read and maintain compared to using Promises. It makes the code appear synchronous, simplifies logic, and eases error handling.